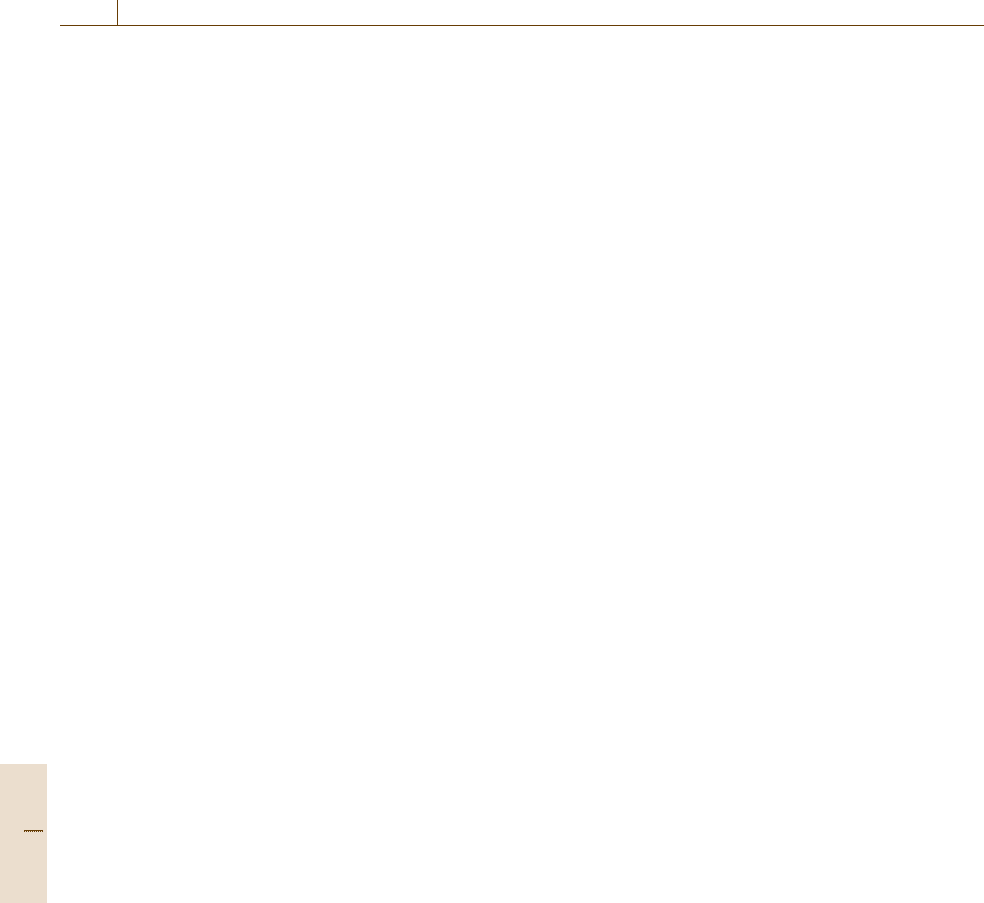
252 Part B Automation Theory and Scientific Foundations
search procedure [14.6] is a special case of best-first
search, with some modificationsto handle situations
where there are multiple paths to the same state.
Best-first search has the advantage that, if it chooses
an obviously bad state s to explore next, it will not
spend much time exploring the subtree below s.As
soon as it reaches successors of s whose f -values
exceed those of other states on the Active list, best-
first search will go back to those other states. The
biggest drawback is that best-first search must re-
member every state it has ever visited, hence its
memory requirement can be huge. Thus, best-first
search is more likely to be a good choice in cases
where the state space is relatively small, and the dif-
ficulty of solving the problem arises for some other
reason (e.g., a costly-to-compute heuristic function,
as in [14.7]).
•
In depth-first branch and bound, at line 4 the algo-
rithm always chooses the longest path in Active;
if there are several such paths then the algorithm
chooses the one that has the smallest value for f(π).
The algorithm maintains a variable π
∗
that holds
the best solution seen so far, and the pruning step
in line 7 removes a path π iff f (π) ≥ F(π
∗
). If the
state space is finite and acyclic, at least one solu-
tion exists, and (14.1) holds, then depth-first branch
and bound is guaranteed to return a solution π
∗
that
minimizes F(π
∗
).
The primary advantage of depth-first search is its
low memory requirement: the number of nodes in
Active will never exceed bd, where d is the length
of the current path. The primary drawback is that, if
it chooses the wrong state to look at next, it will
explore the entire subtree below that state before
returning and looking at the state’s siblings. Depth-
first search does better in cases where the likelihood
of choosing the wrong state is small or the time
needed to search the incorrect subtrees is not too
great.
•
Greedy search is a state-space search without
any backtracking. It is accomplished by replac-
ing line 8 with Active ←{π
1
}, where π
1
is the
path in Successors that minimizes { f (π
) | π
∈
Successors}. Beam search is similar except that, in-
stead of putting just one successor π
1
of π into
Active, we put k successors π
1
,...,π
k
into Active,
for some fixed k.
Both greedy search and beam searchwill return very
quickly once they find a solution, since neither of
them will spend any time looking for better solu-
tions. Hence they are good choices if the state space
is large, most paths lead to solutions, and we are
more interested in finding a solution quickly than in
finding an optimal solution. However, if most paths
do not lead to solutions, both algorithms may fail to
find a solution at all (although beam search is more
robust in this regard, since it explores several paths
rather than just one path). In this case, it may work
well to do a modified greedy search that backtracks
and tries a different path every time it reaches a dead
end.
Hill-Climbing
A hill-climbing problemis a specialkind of search prob-
lem in which every state is a goal state. A hill-climbing
procedure is like a greedy search, except that Active
contains a single state rather than a single path; this
is maintained in line 6 by inserting a single successor
of the current state s
k
into Active, rather than all of
s
k
’s successors. In line 5, the algorithm terminates when
none of s
k
’s successors looks better than s
k
itself, i.e.,
when s
k
has no successor s
k+1
with f (s
k+1
) > f(s
k
).
There are several variants of the basic hill-climbing ap-
proach:
•
Stochastic hill-climbing and simulated annealing.
One difficulty with hill-climbing is that it will ter-
minate in cases where s
k
is a local minimum but not
a global minimum. To prevent this from happening,
a stochastic hill-climbing procedure does not always
return when the test in line 5 succeeds. Probably the
best known example is simulated annealing, a tech-
nique inspired by annealing in metallurgy, in which
a materialis heated and then slowly cooled. In simu-
lated annealing, this is accomplished as follows. At
line 5, if none of s
k
’s successors look better than s
k
then the procedure will not necessarily terminate as
in ordinary hill-climbing; instead it will terminate
with some probability p
i
, where i is the number of
loop iterations and p
i
grows monotonically with i.
•
Genetic algorithms. A genetic algorithm is a mod-
ified version of hill-climbing in which successor
states are generated not using the normal successor
function, but instead using operators reminiscent of
genetic recombination and mutation. In particular,
Active contains k states rather than just one, each
state is a string of symbols, and the operators O are
computational analogues of genetic recombination
and mutation. The termination criterion in line 5 is
generally ad hoc; for example, the algorithm may
terminate after a specified number of iterations, and
return the best one of the states currently in Active.
Part B 14.1