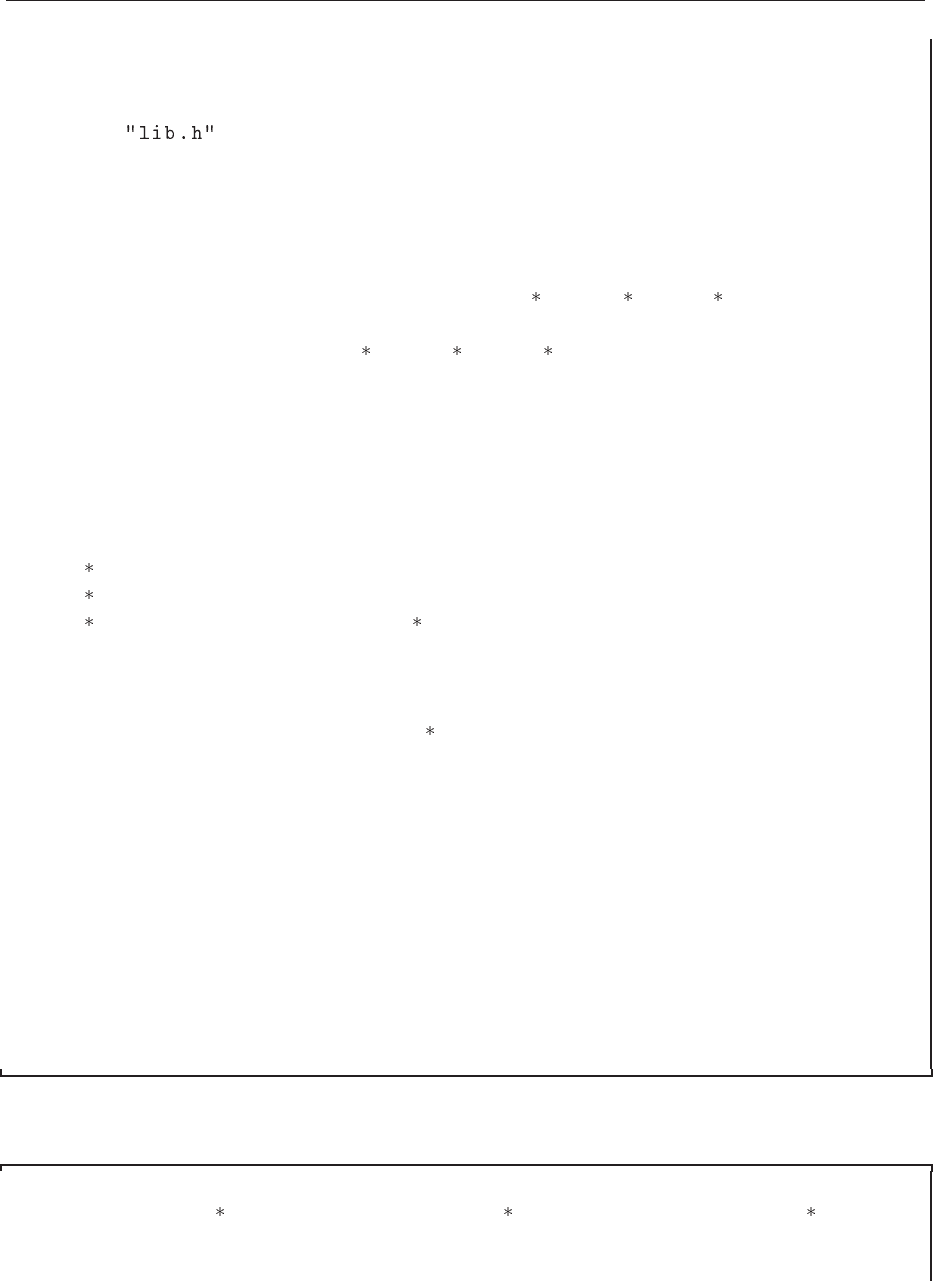
178 CHAPTER 10. RANDOM WALKS AND THE METROPOLIS ALGORITHM
#include < iostream >
#include < fstream >
#include < iomanip >
#include
using namespace std ;
/ / Function to read in data from screen , note c a ll by reference
void i n i t i a l i s e ( int & , int & , double&) ;
/ / The Mc sampling for random walks
void mc_sampling ( int , int , double , int , int , int ) ;
/ / p r int s to screen the r e s u l t s of the c a lcu l at i ons
void output ( int , int , int , int , int ) ;
int main ()
{
int max_trials , number_walks ;
double move_probability ;
/ / Read in data
i n i t i a l i s e ( max_trials , number_walks , move_probability ) ;
int walk_cumulative = new int [ number_walks +1];
int walk2_cumulative = new int [ number_walks +1];
int pr o b a b i lit y = new int [ 2 ( number_walks +1) ] ;
for ( int walks = 1 ; walks <= number_walks ; walks ++){
walk_cumulative [ walks ] = walk2_cumulative [ walks ] = 0 ;
}
for ( int walks = 0 ; walks <= 2 number_walks ; walks ++){
prob abi l i t y [ walks ] = 0 ;
} / / end i n i t i a l i z a t i o n of vectors
/ / Do the mc sampling
mc_sampling ( max_trials , number_walks , move_probability ,
walk_cumulative , walk2_cumulative , p r o b a bil i ty ) ;
/ / Print out re s u l t s
output ( max_trials , number_walks , walk_cumulative ,
walk2_cumulative , p roba b i l i t y ) ;
delete [ ] walk_cumulative ; / / fr ee memory
delete [ ] walk2_cumulative ; delete [ ] p r o b abili t y ;
return 0 ;
} / / end main f un ct io n
The output function contains now the normalization of the probability as well and writes this to
its own file.
void output ( int max_trials , int number_walks ,
int walk_cumulative , int walk2_cumulative , int
p r obab i l i ty )
{