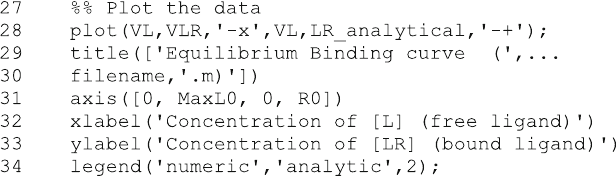
subroutine function 'EQ1F' where the equations are written. Note that the
name'EQ1F' is a string of characters as defined by single-quote ('). fsolve
also requires the vector x0 of initial estimates as an argument. The result in line 20
is the vector x. In lines 21 and 22, the elements of the vector x are translated into
the chemical notation as free receptor R and free ligand L, respectively. Line 23
defines VLR as the vector of complexes LR, employing (2.15). VL (line 24 ) simply
gives the vector of free ligand concentration L. The loop ends with an end
statement. Octave allows different end statements like endfor, endif,
endwhile and endfunction. Since MATLAB does not support this, we do
not make use of this Octave feature.
The loop creates the vectors VLR and VL of complex and free ligand, respec-
tively. Calculating the analytical solution in octave does not require a loop. Line 26
is octave code and looks identical to (3.1), but note that VL is a vector of
concentrations L. In line 26 all concentrations of bound ligand LR for all free
ligand concentrations L are calculated with one statement. The operator ./ (dot
followed by forward slash) in line 26 denot es an element-by-element division.
It corresponds to the element-by-element multiplication explained in Sect. 4.7.
Once all relevant concentrations are calculated, they can be plotted with the plot
command given in line 28. The first argument in the plot function is the x-value, in
our case the free concentration vector VL and the second is the corresponding y-
value, the bound ligand vector VLR. The style (included in quotes) specifies line ()
and symbol (x). A second pair of x and y coordinates is supplied for the analytical
solution (LR_analytical). This is specified by another symbol (+). Lines 29 and 30
are remarkable for two reasons. First, the continuation marker (three dots) at the end
of a line indicates that the next line belongs to the same statement. In this case, lines
29 and 30 are pasted to a single title function. The second remarkable feature of
the
title function is the string which is given as an argument. Every string may be
regarded as a row vector of characters. In Octave, any row vector is defined by
its elements, separated by commas, in square brackets (Sect. 4.7). Therefore
['Equilibrium Binding curve (',filename,'.m)'] is a row vector of
the string 'Equilibrium Binding curve (', followed by the parameter string
filename (defined in line 8), followed by the string '.m)'.
The same procedure (row vector of strings) is used in the print and save
commands in lines 36, 37, 38 and 40. Unfortunately, Octave version 3.2.4 gives
a message implicit conversion from matrix to string whenever a
print command (line 36 and 37) is executed. Such a warning is not given when the
5.2 Equilibrium Binding to One Site (EQ1.m) 47