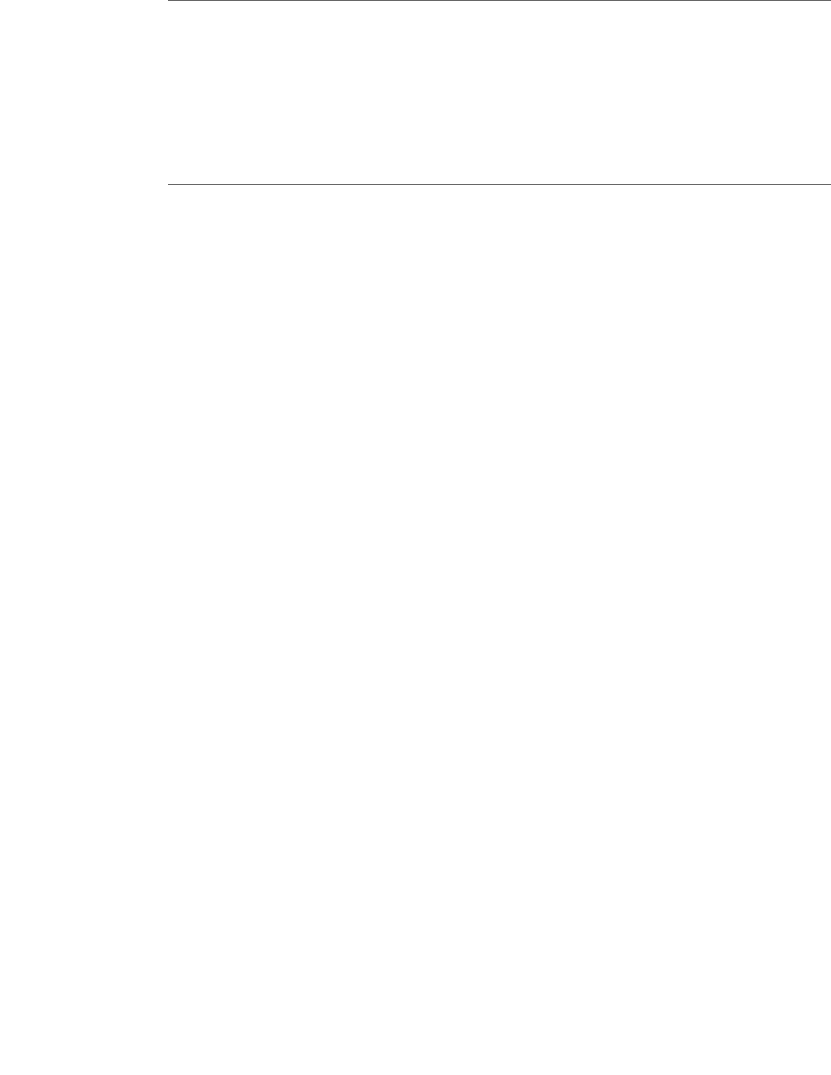
Section 15.1 Chapter 15 · Case Classes and Pattern Matching 296
def simplifyTop(expr: Expr): Expr = expr match {
case UnOp("-", UnOp("-", e)) => e // Double negation
case BinOp("+", e, Number(0)) => e // Adding zero
case BinOp("
*
", e, Number(1)) => e // Multiplying by one
case _ => expr
}
Listing 15.2 · The simplifyTop function, which does a pattern match.
The right-hand side of simplifyTop consists of a match expression.
match corresponds to switch in Java, but it’s written after the selector ex-
pression. I.e., it’s:
selector match { alternatives }
instead of:
switch (selector) { alternatives }
A pattern match includes a sequence of alternatives, each starting with the
keyword case. Each alternative includes a pattern and one or more expres-
sions, which will be evaluated if the pattern matches. An arrow symbol =>
separates the pattern from the expressions.
A match expression is evaluated by trying each of the patterns in the
order they are written. The first pattern that matches is selected, and the part
following the arrow is selected and executed.
A constant pattern like "+" or 1 matches values that are equal to the
constant with respect to ==. A variable pattern like e matches every value.
The variable then refers to that value in the right hand side of the case clause.
In this example, note that the first three examples evaluate to e, a variable
that is bound within the associated pattern. The wildcard pattern (_) also
matches every value, but it does not introduce a variable name to refer to that
value. In Listing 15.2, notice how the match ends with a default case that
does nothing to the expression. Instead, it just results in expr, the expression
matched upon.
A constructor pattern looks like UnOp("-", e). This pattern matches
all values of type UnOp whose first argument matches "-" and whose sec-
ond argument matches e. Note that the arguments to the constructor are
Cover · Overview · Contents · Discuss · Suggest · Glossary · Index