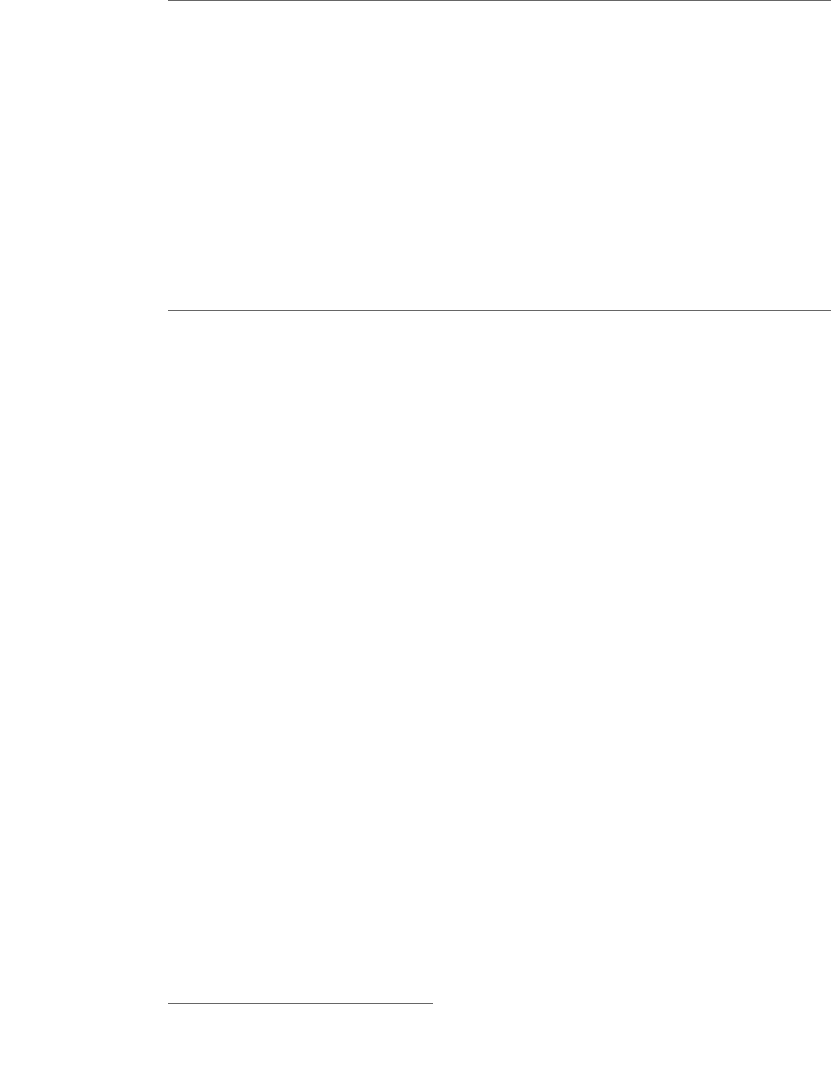
Section 14.7 Chapter 14 · Assertions and Unit Testing 290
import org.scalatest.junit.JUnit3Suite
import org.scalatest.prop.Checkers
import org.scalacheck.Prop._
import Element.elem
class ElementSuite extends JUnit3Suite with Checkers {
def testUniformElement() {
check((w: Int) => w > 0 ==> (elem('x', w, 3).width == w))
check((h: Int) => h > 0 ==> (elem('x', 2, h).height == h))
}
}
Listing 14.10 · Checking properties from a JUnit TestCase with Checkers.
Test’s Checkers trait makes this easy. Simply mix Checkers into your test
class, and pass properties to one of several “check” methods. For example,
Listing 14.10 shows a JUnit3Suite performing the same two ScalaCheck
property checks shown in the previous example, but this time in a single test.
As with all JUnit3Suites, this class is a JUnit TestCase and can therefore
be run with either ScalaTest or JUnit.
4
14.7 Organizing and running tests
Each framework mentioned in this chapter provides some mechanism for
organizing and running tests. In this section, we’ll give a quick overview
of ScalaTest’s approach. To get the full story on any of these frameworks,
however, you’ll need to consult their documentation.
In ScalaTest, you organize large test suites by nesting Suites inside
Suites. When a Suite is executed, it will execute its nested Suites as
well as its tests. The nested Suites will in turn execute their nested Suites,
and so on. A large test suite, therefore, is represented as a tree of Suite
objects. When you execute the root Suite in the tree, all Suites in the tree
will be executed.
You can nest suites manually or automatically. To nest manually, you ei-
ther override the nestedSuites method on your Suites, or pass the Suites
you want to nest to the constructor of class SuperSuite, which ScalaTest
4
You can download ScalaCheck from http://code.google.com/p/scalacheck/.
Cover · Overview · Contents · Discuss · Suggest · Glossary · Index