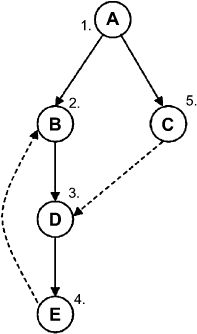
80 Holger Schlingloff
Here we assume that all nodes are “unmarked” in the beginning. The
depth-first search begins when the procedure depth-first-search(x
1
)is
started for any node x
1
.Ifx
1
is followed by the nodes y
1
, y
2
, y
3
, etc., depth-
first-search(y
1
), depth-first-search(y
2
), and so on, will be started in
order. However, if for example y
2
has the successor nodes z
1
, z
2
, etc., then
depth-first-search(z
1
), depth-first-search(z
2
), and so on, will be com-
pleted for all z before depth-first-search for y
3
is started. If the search
leads to a node that doesn’t have any successors (a dead end), or a node that
has already been marked, the search is not continued, but will return to the
previous node, and so on.
For our jungle scenario this means that at an intersection you drop a
pebble and then systematically test all possible paths which start from this
intersection. If you reach a dead end at one path, or see a pebble lying on
the ground that you know you dropped before, you return to the previous
intersection and try another path from there. This makes more sense than
just randomly following any path and then, if it reaches a dead end or be-
comes cyclic, returning all the way to the starting point to try a different
path.
This picture illustrates the algorithm for our sec-
ond scenario, in which Andy is trying in vain to
catch a movie with his friends Benny and Charly.
The nodes (which here represent the people in-
volved) are drawn in order from top to bottom (from
A to E); the numbers next to the nodes show the
order in which the nodes are reached and marked by
the algorithm. The search starts with node A, and
therefore with the call depth-first-search(A).
Since A is not marked yet, depth-first-search(B)
and depth-first-search(C) are started in order.
depth-first-search(C) will run after depth-first-
search(B) and depth-first-search of all suc-
cessors of B are completely finished. depth-first-
search(B) calls depth-first-search(D), which
calls depth-first-search(E), which calls depth-
first-search(B), since B is a successor of E. Node B, however, is already
marked; therefore, we go back to the previous node, E. This node does not
have any other successors than B. Therefore, we return to D, then to B, and
then to A. Here we see that A actually has another successor that has not
been called yet, C; therefore, depth-first-search(C) is started now. The
only successor of C, node D, is already marked, so we return to C and then
to A. Now all calls are finished and the algorithm is done.
When trying to find cycles in graphs, the goal is to discover whether
or not a graph contains any cycles, and, if possible, have one (or some) of