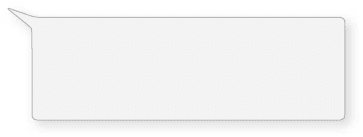
void draw() {
// Get the overall volume (between 0 and 1.0)
float vol = LiveInput.getLevel();
// If the volume is greater than 0.5, draw a rectangle
if (vol > 0.5) {
stroke(0);
fill(0,100);
rect(random(width),random(height),vol*20,vol*20);
}
// Graph the overall volume
// First draw a background strip
fill(175);
rect(0,0,20,height);
// Then draw a rectangle size according to volume
fill(0);
rect(0,height-vol*height/2,20,vol*height/2);
}
// Close the sound engine
public void stop() {
Sonia.stop();
super.stop();
}
is application works fairly well, but does not truly emulate the clapper. Notice how each clap results in
several rectangles drawn to the window. is is because the sound, although seemingly instantaneous to
our human ears, occurs over a period of time. It may be a very short period of time, but it is enough to
sustain a volume level over 0.5 for several cycles through draw( ) .
In order to have a clap trigger an event one and only one time, we need to rethink the logic of our
program. In plain English, this is what we are trying to achieve:
• If the sound level is above 0.5, then you are clapping and trigger the event. However, do not trigger the
event if you just did a moment ago!
e key here is how we defi ne “ a moment ago. ” One solution would be to implement a timer, that is, only
trigger the event once and then wait one second before you are allowed to trigger the event again. is is
a perfectly OK solution. Nonetheless, with sound, a timer is totally unnecessary since the sound itself will
tell us when we are fi nished clapping!
• If the sound level is less than 0.25, then it is quiet and we have finished clapping.
OK, with these two pieces of logic, we are ready to program this “ double-thresholded ” algorithm. ere
are two thresholds, one to determine if we have started clapping, and one to determine if we have
fi nished. We will need a boolean variable to tell us whether we are currently clapping or not.
Assume clapping false to start.
• If the sound level is above 0.5 and we are not already clapping, trigger the event and set clapping t r u e .
• If we are clapping and the sound level is less than 0.25, then it is quiet and set clapping false.
394 Learning Processing
If the volume is greater than 0.5
a rectangle is drawn at a random
location in the window. The louder
the volume, the larger the rectangle.