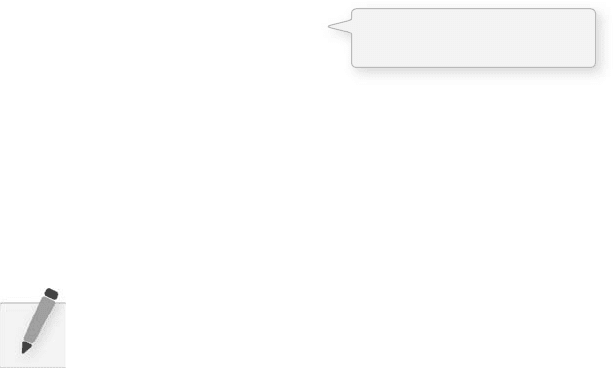
Sound 391
// Pressing the mouse stops and starts the sound
void mousePressed() {
if (tone.isPlaying()) {
tone.stop();
} else {
tone.repeat();
}
}
// Close the sound engine
public void stop() {
Sonia.stop();
super.stop();
}
Exercise 20-4: In Example 20-4, fl ip the Y-axis so that the lower sound plays when the
mouse is down rather than up.
An AudioPlayer object can also be manipulated with Minim using the functions: setVolume( ) ,
setPan( ) , and addEff ect( ) , among others documented on the Minim site ( http://code.compartmental.
net/tools/minim/ ).
20.5 Live input
In Chapter 16, we looked at how serial communication allows a Processing sketch to respond to input
from an external hardware device connected to a sensor. Reading input from a microphone is a similar
pursuit. In essence, the microphone acts as a sensor. Not only can a microphone record sound, but it can
determine if the sound is loud, quiet, high-pitched, low-pitched, and so on. For example, a Processing
sketch could determine if it is living in a crowded room based on sound levels, or whether it is listening
to a soprano or bass singer based on pitch levels. is section will cover how to retrieve and use sound
volume data using the Sonia library. For analyzing sound pitch levels from a microphone, visit the Sonia
web site ( http://sonia.pitaru.com ) for further examples.
e previous sections used a Sample object to play a sound. Sound input from a microphone is retrieved
with a LiveInput object. ere is a somewhat odd distinction here in the way we will use these two
classes that we have yet to encounter over the course of this book.
Consider a scenario where we have three sound fi les. We would create three Sample objects.
Sample sample1 = new Sample( "file1.wav");
Sample sample2 = new Sample( "file2.wav");
Sample sample3 = new Sample( "file3.wav");
Technically speaking, we have made three instances of Sample objects, born via the Sample class. If we
want to play a sound, we have to refer to a specifi c Sample object.
sample1.play();
The sound can be stopped
with the function stop().