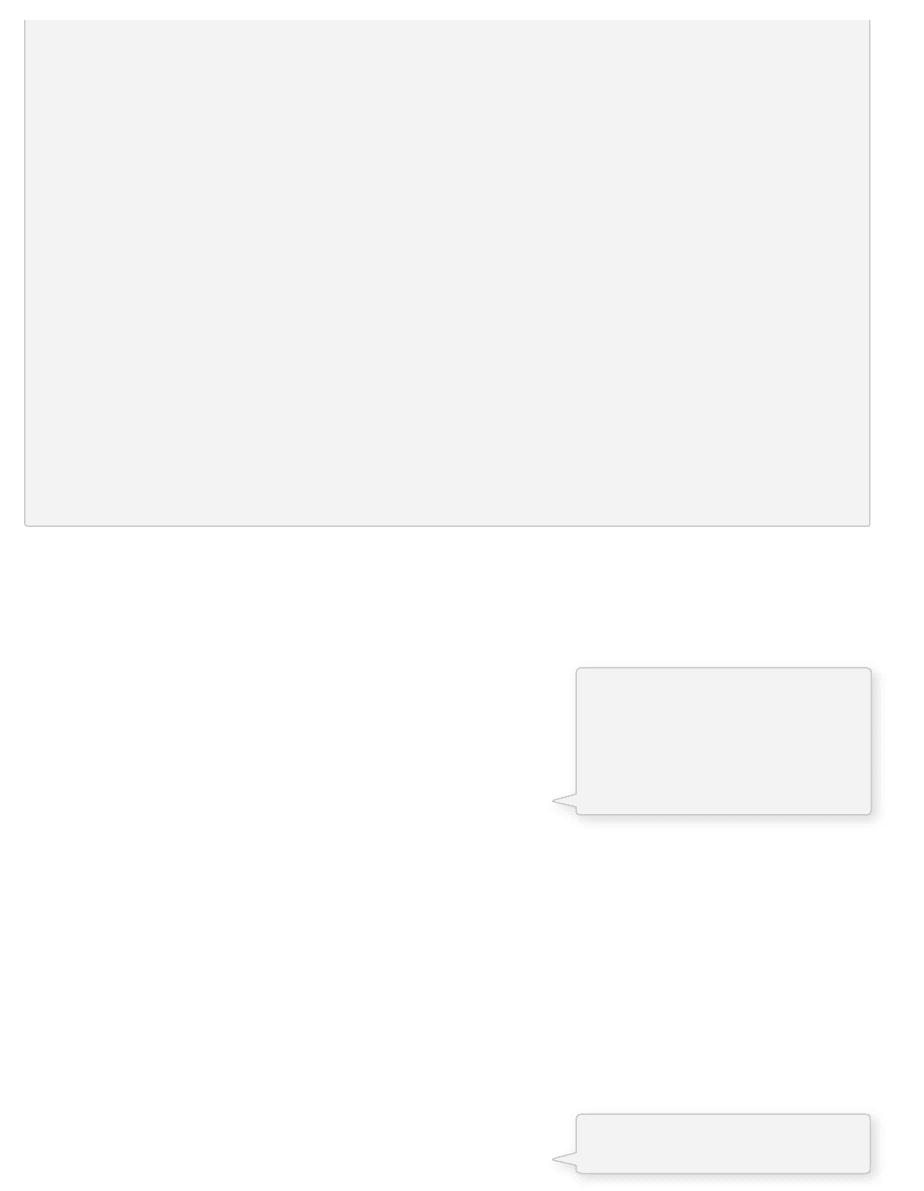
368 Learning Processing
We are now ready to create a server to receive the messages from the client. It will be the client’s job to
format those messages with our protocol. e job of the server remains simple: (1) receive the data and
(2) relay the data. is is similar to the approach we took in Section 19.2.
Step 1 . Receiving data.
Client client = server.available();
if (client ! = null) {
incomingMessage = client. readStringUntil( ' * ' );
}
What is new in this example is the function readStringUntil( ) . e readStringUntil( ) function takes
one argument, a character. at character is used to mark the end of the incoming data. We are simply
following the protocol established during sending. We are able to do this because we are designing both
the server and the client.
Once that data is read, we are ready to add:
Step 2. Relaying data back out to clients.
Client client = server.available();
if (client ! = null) {
incomingMessage = client.readStringUntil( ' * ' );
server.write(incomingMessage);
}
Let’s assume we want to send the number 42. We have two options:
client.write(42); // sending the byte 42
In the line above, we are really sending the actual byte 42.
client.write( " 42 " ); // sending the String " 42 "
In the line above, we are sending a String . at String is made up of two characters, a ‘ 4 ’ and a ‘ 2 ’ .
We are sending two bytes! ose bytes are determined via the ASCII (American Standard Code
for Information Interchange) code, a standardized means for encoding characters. e character ‘ A ’
is byte 65, the character ‘ B ’ 66, and so on. e character ‘ 4 ’ is byte 52 and ‘ 2 ’ is 50.
When we read the data, it is up to us to know whether we want to interpret the bytes coming in
as literal numeric values or as ASCII codes for characters. We accomplish this by choosing the
appropriate read( ) function.
int val ⴝ client.read(); // matches up with client.write(42);
String s ⴝ client.readString(); // matches up with client.write( " 42 " );
int num ⴝ int(s); // convert the String that is read into a number
Because we designed our own
protocol and are not using
newline/carriage return to mark
the end of our message, we must
use readStringUntil() instead of
readString().
Writing the message back out to
all clients.