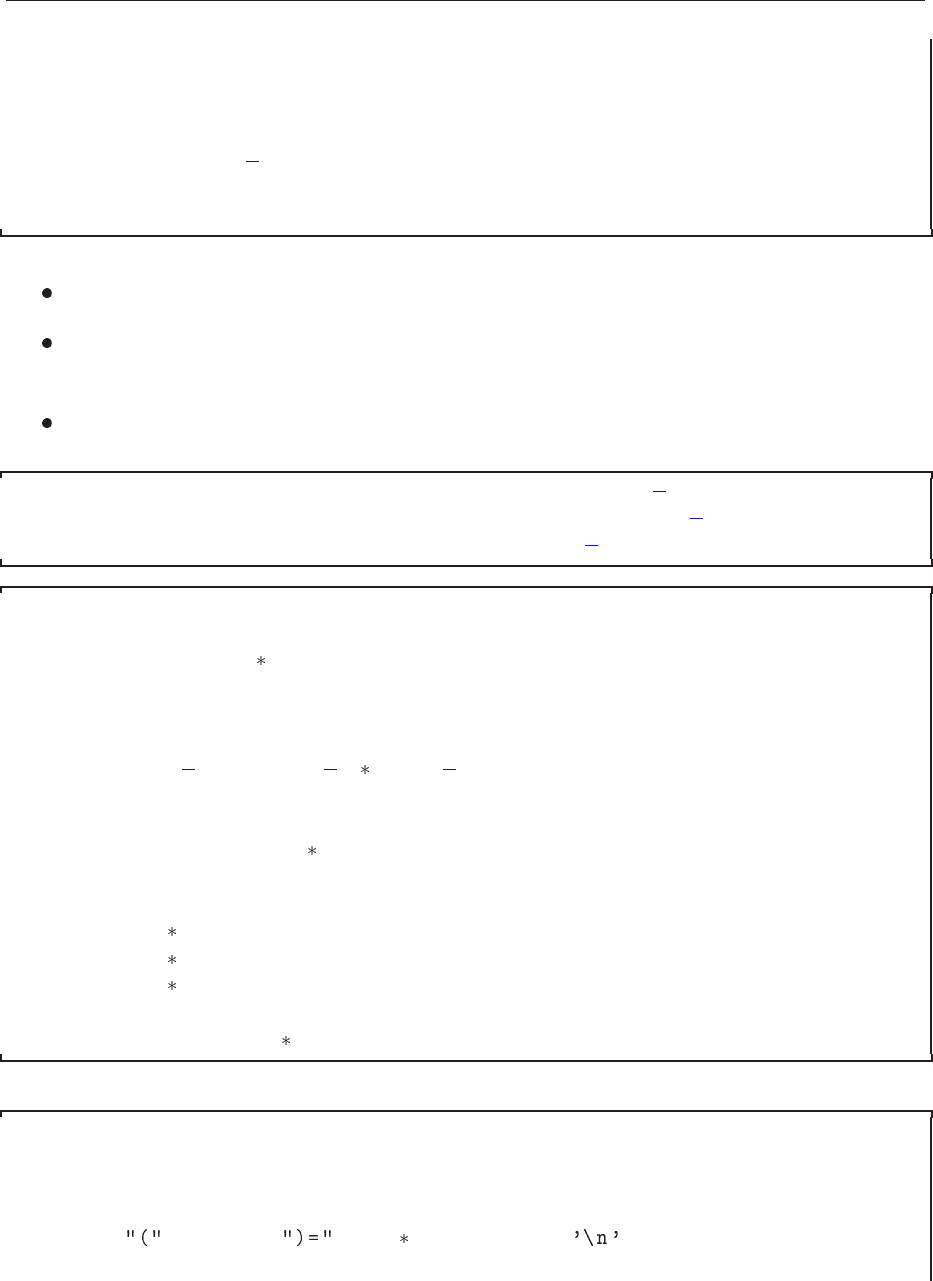
4.2. A FIRST ENCOUNTER, THE VECTOR CLASS 65
/ / the meaning of a ( j ) i s defined by
in line double& MyVector : : operator ( ) ( int i )
{
return A[ i 1];
/ / base index i s 1 ( not 0 as in C/C++)
}
Inline functions: function body is copied to calling code, no overhead of function call!
Note: inline is just a hint to the compiler; there is no guarantee that the compiler really
inlines the function
Why return a double reference?
double& MyVector : : operator ( ) ( int i ) { return A[ i 1]; }
/ / returns a r eference ( ‘ ‘ poi nter ’ ’) d i r e c t l y to A[ i 1]
/ / such that the c all ing code can change A[ i 1]
/ / given MyVector a ( n ) , b(n) , c ( n) ;
for ( int i = 1 ; i <= n ; i ++)
c ( i ) = a ( i ) b ( i ) ;
/ / compiler i n l i n i n g t r a n s l a t e s t h i s to :
for ( int i = 1 ; i <= n ; i ++)
c .A[ i 1] = a .A[ i 1] b .A[ i 1];
/ / or perhaps
for ( int i = 0 ; i < n ; i ++)
c .A[ i ] = a .A[ i ] b .A[ i ] ;
/ / more op ti m iz ation s by a smart compiler :
double ap = & a .A[ 0 ] ; / / s t a r t of a
double bp = &b .A[ 0 ] ; / / s t a r t of b
double cp = & c .A[ 0 ] ; / / s t a r t of c
for ( int i = 0 ; i < n ; i ++)
cp [ i ] = ap [ i ] bp [ i ] ; / / pure C!
Inlining and the programmer’s complete control with the definition of subscripting allow
void MyVector : : p r i n t ( std : : ostream & o ) const
{
int i ;
for ( i = 1 ; i <= length ; i ++)
o < < < < i < < < < ( this ) ( i ) < < ;
}