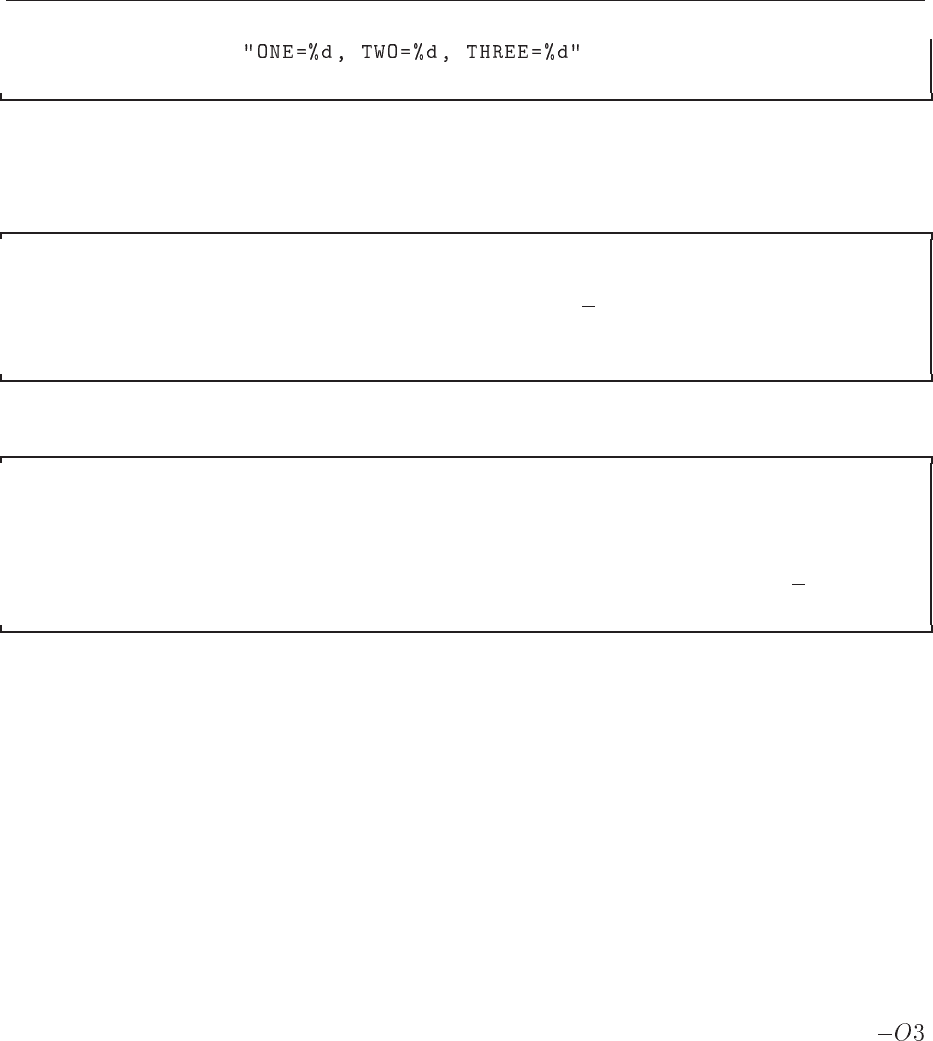
38 CHAPTER 2. INTRODUCTION TO C/C++ AND FORTRAN 90/95
7 . pri n t f ( ,ONE,TWO,THREE) ;
8 . }
In C++ the usage of macros is discouraged and you should rather use the declaration for con-
stant variables. You would then replace a statement like #define ONE 1 with const int ONE = 1;.
There is typically much less use of macros in C++ than in C. Similarly, In C we could define
macros for functions as well, as seen below.
1 . # define MIN( a , b) ( ( ( a ) < ( b) ) ? ( a ) : ( b ) )
2 . # define MAX(a , b ) ( ( ( a ) > ( b ) ) ? ( a ) : ( b ) )
3 . # define ABS( a ) ( ( ( a ) < 0) ? ( a ) : ( a ) )
4 . # define EVEN( a ) ( ( a ) %2 == 0 ? 1 : 0 )
5 . # define TOASCII( a ) ( ( a ) & 0 x7f )
In C++ we would replace such function definitionby employing so-called inline functions. Three
of the above functions could then read
in line double MIN( double a , double b ) ( return ( ( ( a ) < ( b ) ) ? ( a
) : ( b ) ) ; )
in line double MAX( double a , double b ) ( return ( ( ( a ) > ( b ) ) ? ( a
) : ( b ) ) ; )
in line double ABS( double a ) ( return ( ( ( a ) < 0) ? (a ) : ( a )
) ; )
where we have defined the transferred variables to be of type double. The functions also return a
double type. These functions could easily be generalized throughthe use of classes and templates,
see chapter 5, to return whather types of real, complex or integer variables.
Inline functions are very useful, especially if the overhead for calling a function implies a
significant fraction of the total function call cost. When such function call overheadis significant,
a function definition can be preceded by the keyword
inline. When this function is called, we
expect the compiler to generate inline code without function call overhead. However, although
inline functions eliminate function call overhead, they can introduce other overheads. When a
function is inlined, its code is duplicated for each call. Excessive use of
inline may thus generate
large programs. Large programs can cause excessive paging in virtual memory systems. Too
many inline functions can also lengthen compile and link times, on the other hand not inlining
small functions like the above that do small computations, can make programs bigger and slower.
However, most modern compilers know better than programmer which functions to inline or not.
When doing this, you should also test various compiler options. With the compiler option
inlining is done automatically by basically all modern compilers.
A good strategy, recommended in many C++ textbooks, is to write a code without inline
functions first. As we also suggested in the introductory chapter, you should first write a as simple
and clear as possible program, without a strong emphasis on computational speed. Thereafter,
when profiling the program one can spot small functions which are called many times. These
functions can then be candidates for inlining. If the overall time comsumption is reduced due
to inlining specific functions, we can proceed to other sections of the program which could be
speeded up.