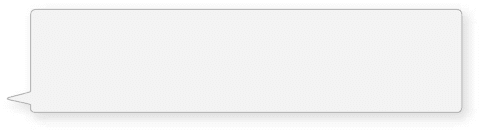
Debugging 193
Once all the code is commented out, there are two possible outcomes. Either the apple still does not
appear or it does. In the former, the issue is most defi nitely caused by the apple itself, and the next step
would be to investigate the insides of the display( ) function and look for a mistake.
If the apple does appear, then the problem is caused by one of the other lines of code. Perhaps the
move( ) function sends the apple off screen so that we do not see it. Or maybe the Snake objects
cover it up by accident. To fi gure this out, I would recommend putting back lines of code, one at a time.
Each time you add back in a line of code, run the sketch and see if the apple disappears. As soon as it
does, you have found the culprit and can root out the cause. Having an object-oriented sketch as above
(with many classes) can really help the debugging process. Another tactic you can try is to create a new
sketch and just use one of the classes, testing its basic features. In other words, do not worry about fi xing
your entire program just yet. First, create a new sketch that only does one thing with the relevant class
(or classes) and reproduce the error. Let’s say that, instead of the apple, the snakes are not behaving
properly. To simplify and fi nd the bug, we could create a sketch that just uses one snake (instead of an
array) without the apple or the button. Without the bells and whistles, the code will be much easier to
deal with.
Snake snake;
void setup() {
size(200,200);
snake = new Snake();
}
void draw() {
background(0);
snakes.display();
snakes.slither();
//snakes.eat(apple);
}
Although we have not yet looked at examples that involve external devices (we will in many of the
chapters that follow), simplifying your sketch can also involve turning off connections to these devices,
such as a camera, microphone, or network connection and replacing them with “ dummy ” information.
For example, it is much easier to fi nd an image analysis problem if you just load a JPG, rather than use
a live video source. Or load a local text fi le instead of connecting to a URL XML feed. If the problem
goes away, you can then say defi nitively: “ Aha, the web server is probably down ” or “ My camera must be
broken. ” If it does not, then you can dive into your code knowing the problem is there. If you are worried
about worsening the problem by taking out sections of code, just make a copy of your sketch fi rst before
you begin removing features.
11.4 Tip #4: println( ) is your friend.
Using the message window to display the values of variables can be really helpful. If an object is
completely missing on the screen and you want to know why, you can print out the values of its location
variables. It might look something like this:
println( "x: " + thing.x + " y: " + thing.y);
Since this version does not include an Apple object,
we cannot use this line of code. As part of the
debugging process, however, we might incrementally
add back in the apple and uncomment this line.