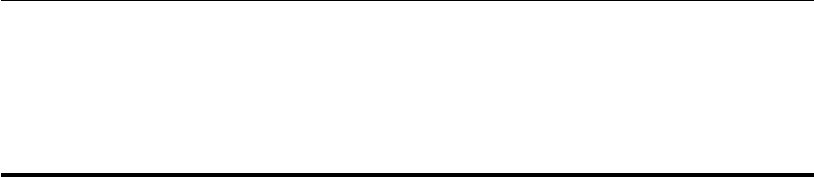
■
3.4 Implementing Wire Formats in C# 71
3.4.1 Text-Oriented Representation
Clearly we can represent the ItemQuote information as text. One possibility is simply to
transmit the output of the ToString() method using a suitable character encoding. To
simplify parsing, the approach in this section uses a different representation, in which
the values of itemNumber, itemDescription, and so on are transmitted as a sequence of
delimited text fields. The sequence of fields is as follows:
Item NumberDescriptionQuantityPriceDiscount?In Stock?
The Item Number field (and the other integer-valued fields, Quantity and Price) contain
a sequence of decimal-digit characters followed by a space character (the delimiter). The
Description field is just the description text. However, because the text itself may include
the space character, we have to use a different delimiter; we choose the newline character,
represented as \n in C#, as the delimiter for this field.
Boolean values can be encoded in several different ways. Although a single-byte
Boolean is one of the overloaded arguments in the BinaryWriter Write() method, in order
to keep our wire format slightly more language agnostic (and to allow it to communicate
with the Java versions of these programs [25]) we opted not to use it. Another possibility is
to include the string “true” or the string “false,” according to the value of the variable. A
more compact approach (and the one used here) is to encode both values (discounted and
inStock) in a single field; the field contains the character ‘d’ if discounted is true, indicating
that the item is discounted, and the character ‘s’ if inStock is true, indicating that the item
is in stock. The absence of a character indicates that the corresponding Boolean is false,
so this field may be empty. Again, a different delimiter (\n) is used for this final field, to
make it slightly easier to recognize the end of the message even when this field is empty.
A quote for 23 units of item number 12345, which has the description “AAA Battery” and
a price of $14.45, and which is both in stock and discounted, would be represented as
12345 AAA Battery\n23 1445 ds\n
Constants needed by both the encoder and the decoder are defined in the ItemQuote-
TextConst interface, which defines “ascii” as the default encoding (we could just as easily
have used any other encoding as the default) and 1024 as the maximum length (in bytes)
of an encoded message. Limiting the length of an encoded message limits the flexibility of
the protocol, but it also provides for sanity checks by the receiver.
ItemQuoteTextConst.cs
0 using System; // For String
1
2 public class ItemQuoteTextConst {
3 public static readonly String DEFAULT_CHAR_ENC = "ascii";
4 public static readonly int MAX_WIRE_LENGTH = 1024;
5 }
ItemQuoteTextConst.cs