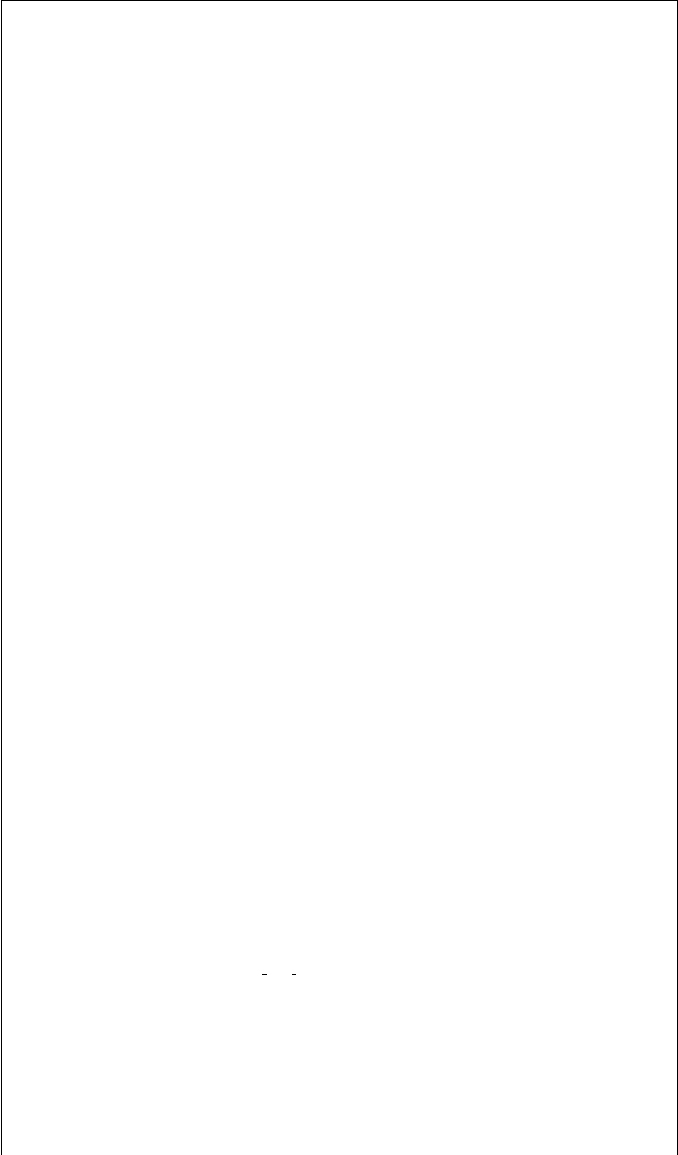
13.2 WMS Server 231
Listing 13.4 Java Servlet implementation of simple WMS server.
1 public class WMSTileServlet extends HttpServlet {
2
3 DataStore tileStorage ;
4
5 public void doGet(HttpServletRequest request , HttpServletResponse response )
{
6 // collect the parameters from the URL
7 String service = request.getParameter(”SERVICE”);
8 // service should equal WMS
9 String version = request. getParameter(”VERSION”);
10 // version should equal 1.1.1
11
12 String requestType = request . getParameter(”REQUEST”) ;
13 if (requestType . equalsIgnoreCase(”GetCapabilities”)) {
14 printCapabilities (request , response ) ;
15 }
16
17 if (requestType . equalsIgnoreCase(”GETMAP”)) {
18 String layers = request.getParameter(”LAYERS”) ;
19 // layers should equal blue marble
20 String srs = request.getParameter(”SRS”) ;
21 // should be equal to EPSG:4326
22
23 String widthStr = request. getParameter(”WIDTH”) ;
24 String heightStr = request . getParameter(”HEIGHT”);
25 int width = Integer . parseInt (widthStr) ;
26 int height = Integer . parseInt ( heightStr);
27 String format = request.getParameter(”FORMAT”) ;
28 // get bounding box from request
29 String bbox = request . getParameter (”BBOX”) ;
30 String bbdata [] = bbox . sp lit ( ” , ”) ;
31 double minx = Double . parseDouble ( bbdata [0]) ;
32 double miny = Double . parseDouble ( bbdata [1]) ;
33 double maxx = Double . parseDouble ( bbdata [2]) ;
34 double maxy = Double . parseDouble ( bbdata [3]) ;
35 BoundingBox imageBounds = new BoundingBox(minx , miny , maxx , maxy) ;
36 // compute scale to use
37 double dpp = (( maxx − minx ) / width + (maxy − miny) / height ) /
2.0;
38 double [] standardScales = TileStandards . zoomLevels ;
39 int maxscale = tileStorage . getMaxScale(”bluemarble”) ;
40
41 int scaleToUse = maxscale;
42 for ( int i = 0; i < ; maxscale; i++) {
43 if (standardScales[i] < dpp) {
44 scaleToUse = i ;
45 break;
46 }
47 }
48 double tileSize = 360.0 / (Math .pow(2 , scaleToUse) ) ;
49 // calculate bounds of image in t il e coordinates
50 long mincol = ( long) Math.max(0 , Math . floor ((minx + 180.0) /
tileSize));
51 long maxcol = ( long ) Math. floor ((maxx + 180.0) / tileSize ) ;
52 long minrow = ( long ) Math.max(0, Math. floor ((miny + 90.0) /
tileSize));
53 long maxrow = ( long ) Math. floor ((maxy + 90.0) / tileSize );
54
55 // iterate over t il e range , retrieve each t il e and draw to new image
56 BufferedImage bi = new BufferedImage ( width , height ,
57 BufferedImage .TYPE INT RGB) ;
58 for ( long r = minrow ; r < ;= maxrow; r ++) {
59 for ( long c = mincol ; c < ;= maxcol ; c++) {
60 // have to check to see if the cluster actually has any data
...
61 TileAddress tileAddress = new TileAddress (r , c , scaleToUse)
;
62
63 BoundingBox tileBounds = tileAddress .getBoundingBox () ;
64 byte [] imageData = tileStorage . getTileImage (”bluemarble” ,
65 scaleToUse , r , c) ;
66