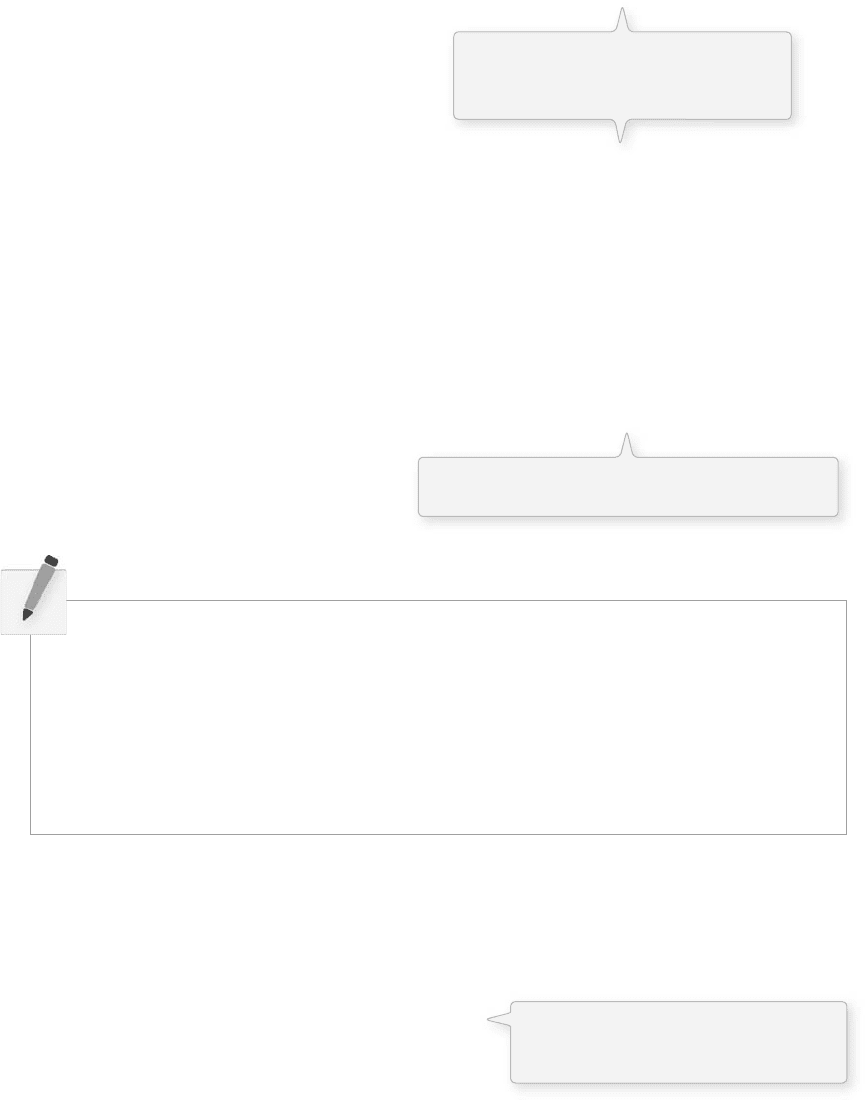
328 Learning Processing
// Splitting a String based on spaces
String spaceswords = " The quick brown fox jumps over the lazy dog. " ;
String[] list = split(spaceswords, " " );
for (int i = 0; i < list.length; i + + ){
println(list[i] + " " + i);
}
Here is an example using a comma as the delimiter.
// Splitting a String based on commas
String commaswords = " The,quick,brown,fox,jumps,over,the,lazy,dog. " ;
String[] list = split(commaswords, ‘ , ’ );
for (int i = 0; i < list.length; i + + ){
println(list[i] + " " + i);
}
If you want to use more than one delimiter to split up a text, you must use the Processing function
splitTokens( ). splitTokens( ) works identically is split( ) with one exception: any character that appears in
the String qualifi es as a delimiter.
// Splitting a String based on multiple delimiters
String stuff = " hats & apples, cars + " ;
String[] list = splitTokens(stuff, " & , + . " );
for (int i = 0; i < list.length; i + + ){
println(list[i] + " " + i);
}
If we are splitting numbers in a String, the resulting array can be converted into an integer array with
Processing ’s int( ) function.
// Calculate sum of a list of numbers in a String
String numbers = " 8,67,5,309 " ;
// Converting the String array to an int array
int[] list = int(split(numbers, ' , ' ));
int sum = 0;
for (int i = 0; i < list.length; i + + ){
sum = sum + list[i];
}
println(sum);
Exercise 18-4: Fill in what the above code will print in the Processing message window:
hats_______________
__________________
__________________
__________________
__________________
This period is not set as a delimeter and
therefore will be included in the last
String in the array: “dog.”
The period is set as a delimiter and therefore will
not be included in the last String in the array: “dog”.
Numbers in a String are not numbers
and cannot be used in mathematical
operations unless we convert them fi rst.
phones % elephants dog.