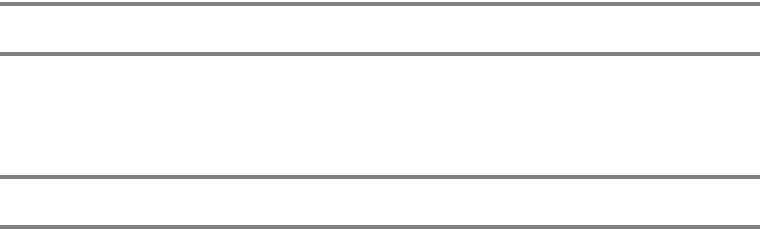
CHAPTER 2 ■ SCALA SYNTAX, SCRIPTS, AND YOUR FIRST SCALA PROGRAMS
27
Scala’s Class Hierarchy
In Scala, everything (except a method) is an instance of a class. This means that Java primitives
such as
int are treated as instances by the Scala compiler. This is done at the compilation
level, but the bytecode is optimized so that adding two
Ints in Scala results in the same
bytecode and performance as adding two
ints in Java. On the other hand, in Scala, Int has
methods including
hashCode and toString. The Scala compiler will put primitive types in a
box when they are being passed to something that expects an
Any, such as putting an Int
in a
HashMap.
In keeping with the naming convention that all classes have uppercase letters at the
beginning of their name, Scala representations of JVM primitives are called
Int, Long,
Double, Float, Boolean, Char, Short, and Byte. They are all subclasses of AnyVal. Scala also has
an object representation of Java’s
void called Unit, which is also a subclass of AnyVal. You
can explicitly return
Unit from a method with the () singleton (yep, you read that right, an
open and a close parenthesis).
scala> val v = ()
v: Unit = ()
scala> List(v)
res1: List[Unit] = List(())
Nothing
is cool. Any method that returns Nothing will never normally return. It must
throw an exception. This makes the
None singleton that we encountered in our sum.scala
program possible.
None is an Option[Nothing], which means its get method returns a Nothing.
This sounds like word games, but it means that the compiler enforces that the
get method
in
None throws an exception rather than returning some other bottom-of-the-hierarchy
value such as
null.
Any is the root of the Scala class hierarchy, like Object is the root of the Java class hier-
archy. But, because of
Nothing, primitives, and so on, Scala needed a root class that is
underneath
Object.
AnyVal is the root of Scala’s objectification of the JVM’s primitives.
AnyRef means the same thing as Java’s Object but has a few extra compiler-managed
methods, including the
eq, ne, == and != methods. == is a call to the equals method rather
than the Java meaning of reference comparison. If you want to do a reference comparison
in Scala, use the
eq method.
19897ch02.fm Page 27 Wednesday, April 1, 2009 5:34 PM