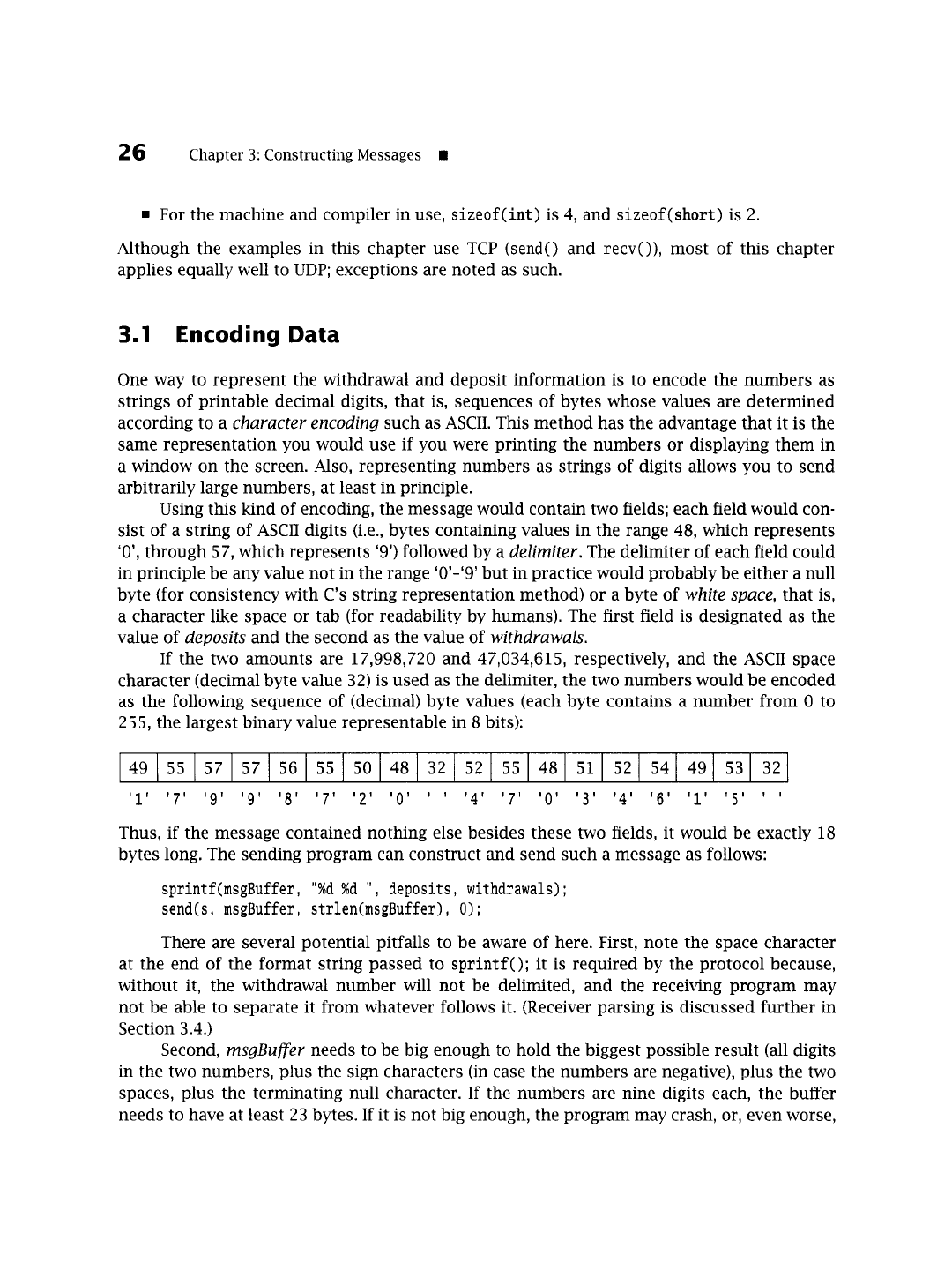
26
Chapter 3: Constructing Messages m
9 For the machine and compiler in use, sizeof(int) is 4, and sizeof(short) is 2.
Although the examples in this chapter use TCP (send() and recv()), most of this chapter
applies equally well to UDP; exceptions are noted as such.
3.1 Encoding Data
One way to represent the withdrawal and deposit information is to encode the numbers as
strings of printable decimal digits, that is, sequences of bytes whose values are determined
according to a
character encoding
such as ASCII. This method has the advantage that it is the
same representation you would use if you were printing the numbers or displaying them in
a window on the screen. Also, representing numbers as strings of digits allows you to send
arbitrarily large numbers, at least in principle.
Using this kind of encoding, the message would contain two fields; each field would con-
sist of a string of ASCII digits (i.e., bytes containing values in the range 48, which represents
'0', through 57, which represents '9') followed by a
delimiter.
The delimiter of each field could
in principle be any value not in the range '0'-'9' but in practice would probably be either a null
byte (for consistency with C's string representation method) or a byte of
white space,
that is,
a character like space or tab (for readability by humans). The first field is designated as the
value of
deposits
and the second as the value of
withdrawals.
If the two amounts are 17,998,720 and 47,034,615, respectively, and the ASCII space
character (decimal byte value 32) is used as the delimiter, the two numbers would be encoded
as the following sequence of (decimal) byte values (each byte contains a number from 0 to
255, the largest binary value representable in 8 bits):
1491 5 1 71 71561551501481321 21551481 511 521 491 s31 321
'2' '7' '9' '9' '8' '7' '2' '0' ' ' '4' '7' '0' '3' '4' '6' '1' '5' ' '
Thus, if the message contained nothing else besides these two fields, it would be exactly 18
bytes long. The sending program can construct and send such a message as follows:
sprintf(msgBuffer,
"%d %d ", deposits, withdrawals) ;
send(s,
msgBuffer, strlen(msgBuffer),
0);
There are several potential pitfalls to be aware of here. First, note the space character
at the end of the format string passed to sprintf(); it is required by the protocol because,
without it, the withdrawal number ~I| not be delimited, and the receiving program may
not be able to separate it from whatever follows it. (Receiver parsing is discussed further in
Section 3.4.)
Second,
msgBuffer
needs to be big enough to hold the biggest possible result (all digits
in the two numbers, plus the sign characters (in case the numbers are negative), plus the two
spaces, plus the terminating null character. If the numbers are nine digits each, the buffer
needs to have at least 23 bytes. If it is not big enough, the program may crash, or, even worse,